Introduction
Code documentation is important to be followed as the standard practise. Obviously its not an interesting job to the code developers, for many reasons and also because of laziness issues. It again depends on the importance of documentation. Lets forget these documentation talks. But bottomline, it is really important for future reference purpose. You will believe one day and some of you already believe that.
Most of the devlepers follow the basic comenting. But if you really want to follow the authentic professional method, .net has it all. The line or block of code is generally commented using //(double forward slash - single line), or /* */ (within slash, asterik block - multiple lines).
But if you want to distribute your code or class libraries to your clients, its very helpful if you submit thorough documentation file along with it for reference purpose.
XML comment tags used for documentation
Lets get into the actuals now. If you type /// (3 slashes) in the source code unit, you will see an XML block that gets auto-generated for you. This is the comment block in xml format. The basic comment tags that show up depends upon where you have put the slashes. If it is above the function block, you will see the below segment,
Sample with default tags
/// <summary>
///
/// </summary>
/// <param name="number1"></param>
/// <param name="number2"></param>
/// <returns></returns>
static int addTwoNumbers(int number1, int number2)
{
}
As seen above, we have <summary>, <param>, <returns> tags added automatically. The <summary> tag is used to provide the summary details on what the method does. The <param> tags are included, to write details on the parameters being passed to the method. And the <returns> is included for details on the return type and/or value from the method.
Note, these xml tags must conform the xml rules otherwise you will get warnings and also you final xml documentation file would not contain the errorneous tags until you fix them.
Lets see other commonly used xml tags available for comments as below:
1. <remarks> - Used to provide detailed information about a type or member.
2. <paramref> - Used to create a reference to a parameter.
3. <see cref="" /> - Used to create a reference to any type or a memeber.
4. <exception cref="" /> - Used to create details on exceptions.
5. <c> or <code> - <c> is Used to demonstrate a
small code segment whereas <code> tag is used to demonstrate multi-lines code segment.
6. <example> - used to demonstrate code examples. Usually the <c> and <code> tags are contained withing <example> tag.
Once these tags are in place in the code, follow these 2 steps and C# compiler will generate the XML Documentation file at the specified location.
a. Check the XML Documentation file checkbox and specify the file location, in the Build tab of the project's properties.
b. Build the application.
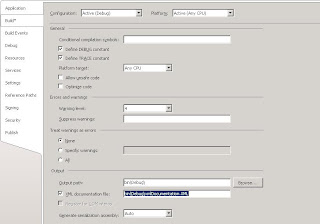
Lets see a complete code source example with some of the comments tags as mentioned above. After the source code is the XML Documentation file generated.
Source Code with XML Comments as Code documentation
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace xmlDocumentation
{
class Program
{
/// <summary>
/// This is the entry point method of this project.
/// </summary>
/// <param name="args"></param>
static void Main(string[] args)
{
int number1 = 1;
int number2 = 2;
addTwoNumbers(number1, number2);
}
/// <summary>
///
/// </summary>
/// <param name="number1"></param>
/// <param name="number2"></param>
/// <returns><see cref="int" /></returns>
/// <paramref name="number1">Number 1 is the big number</paramref>
/// <remarks></remarks>
/// <example>
/// <c>Result = number1+number2;</c>
/// </example>
static int addTwoNumbers(int number1, int number2)
{
return number1 + number2;
}
}
}
XMLDocumentation.xml file
<?xml version="1.0"?>
<doc>
<assembly>
<name>xmlDocumentation</name>
</assembly>
<members>
<member name="M:xmlDocumentation.Program.Main(System.String[])">
<summary>
This is the entry point method of this project.
</summary>
<param name="args"></param>
</member>
<member name="M:xmlDocumentation.Program.addTwoNumbers(System.Int32,System.Int32)">
<summary>
</summary>
<param name="number1"></param>
<param name="number2"></param>
<returns><see cref="T:System.Int32"/></returns>
<paramref name="number1">Number 1 is the big number</paramref>
<remarks>This method will add the two numbers being passed as the parameters and returuns the sum of two numbers as the result.</remarks>
<example>
<c>Result = number1+number2;</c>
</example>
</member>
</members>
</doc>
Representation
This article is mainly focussed on getting you familiarized with the available xml tags that can be used to document your code and generate xml documentation file.
Once your xml documentation .xml file is ready, you need to present it in a common man readable format. Some of the available tools that can be used to convert the xml document are stated below. We suggest you to explore them and use based on your liking and requirements.
a. XSL -
You can develop your own XSL stylesheet to decorate the above .xml comment file.
b. NDoc -
This is an extensible code documentation generation tool for .NET Developers. It is completely free to download and use. It converts the .xml file as above and coverts it into .chm file. It is very easy to use. You can find the download link at: http://sourceforge.net/projects/ndoc/
c. Sandcastle -
Sandcastle is provided by Microsoft. It produces accurate, MSDN Style, comprehensive documentation and is also easy to use. This tool is available for download at: http://www.microsoft.com/downloads/details.aspx?FamilyId=E82EA71D-DA89-42EE-A715-696E3A4873B2&displaylang=en
d. DOxygen -
Another souce code documentation generator tool. It is developed under Linux and Mac OS x but highly portable. It supports many output format but the popular one is HTML that is widely accepted. This tool is also easy and available for free at: http://www.stack.nl/~dimitri/doxygen/download.html#latestsrc
These are some of the common available options. There may be other tools available or may come in future.
Hope you start documenting your code after knowing the available easy and neat options to generate professional documentation. Keep patience and have fun.
0 comments
Post a Comment