MSMQ is a wonderful, easy and powerful methods by which messages can be stored and retrieved from an independent location. This store is unlike databases or a file server where objects can be sent and received independently. This article explains the basics of installing and configuring MSMQ followed by message send and recieve process using an example. MSMQ is further vast in itself. At the end of this article reference sites are available for more information on MSMQ.
What is MSMQ? How it works?
MSMQ is a message queuing system that enables applications to send and receive messages (objects). It is a seperate independent location where messages are stored and retrieved by applications. The receiving application need not be connected at the time when the sender is storing the messages. Messages can be received at a later time when the sender has finished its processing.
Messages can be an xml document, text, images, or in any other format that the receiving application understands. Message Queues can be private or public. Private queues limit their access to the local computer. Public queues are published is the active directory and can be found and accessed by computers in the network.
Before the applications can use the queues for message exchange, they need to be created first. You can use either create them manually or programmatically.
Lets us first see how to install and configure MSMQ. After we install and configure the queue, we will see how to send and receive messages to and from the queue with a good example.
Configuring Messaging Queue:
1. Go to 'Add or Remove programs' through Control Panel.
2. Click on 'Add/Remove Windows Components'.
3. Search for 'Application Server' & click on Details button.
4. Check mark 'Messaging Queue' and hit OK and follow the wizard till the end.
The location for 'Messaging Queue' may be different on your box, just search for 'Messaging Queue', it must exist somewhere in 'Add/Remove Windows Components' window.
Once it is installed, you should see 'Message Queuing' under 'Services and Applications' in Computer Management snap-in as below.
Creating Queues:
Queues can be created manually or programmatically.
Manual Approach:
1. Open the computer management snap-in.
2. Go to the 'Message Queuing' under the 'Services and Applications'.
3. Right click on the 'Private Queues' -> New -> Private Queue. Enter queue name and hit OK. Your queue gets created.
Programmatic Approach:
.Net provides System.Messaging namespace that contains MSMQ related classes.
MessageQueue msgQueue = MessageQueue.Create(@".\Private$\MyQueue");
Now as we know how to create queues, lets see how to send & retrieve to/from our created queue.
As an example, lets create an XML file. Then create a sender application to read and send the XML file to our queue and a receiving application to read and process the XML file.
XML File:
Lets name this xml file to msg.xml, its content as below.
<?xml version="1.0" encoding="utf-8" ?>
<topic>
<name>MSMQ</name>
</topic>
Sender Application:
This application reads the XML file into XMLDocument object (System.XML namespace), copies the Xml into the message body, serializes it using XmlMessageFormatter, and sends it to the Message Queue.
as message to our queue.
static void Main(string[] args)
{
//Create a private queue
MessageQueue msgQueue = MessageQueue.Create(@".\Private$\MyQueue");
//Loads xml into XMLDocument object
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(@"d:\msg.xml");
//Get the xml into the message body
Message msg = new Message();
msg.Body = xmlDoc;
//serialize the message before sending it to the queue
msg.Formatter = new System.Messaging.XmlMessageFormatter();
msgQueue.Send(msg, "msg1");
}
After executing this code, you will see the msg1 created in the queue as below.
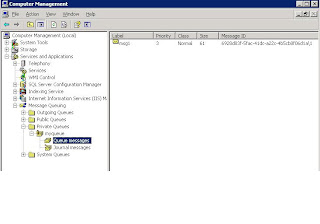
Receiving Application:
As we serialized the message before sending to the queue, at the receiving end the message would need to be deserialized after receiving it and before we could process it further.
static void Main(string[] args)
{
//create & define the message queue
MessageQueue msgQueue = new MessageQueue();
msgQueue.Path = @".\private$\MyQueue";
//Receive the message from the queue.
Message msg = new Message();
msg = msgQueue.Receive();
//define the type of the message being received to deserialize it in the same format as sent
Type[] targettype = new Type[1];
targettype[0] = typeof(XmlDocument);
msg.Formatter = new System.Messaging.XmlMessageFormatter(targettype);
//get the xml message in the XMLDocument object and further process it
XmlDocument xmldoc = new XmlDocument();
xmldoc = (XmlDocument)msg.Body;
Console.Write(xmldoc.InnerXml);
Console.Read();
}
The above example shows how to retrieve a single message. If you want to receive more then one message you can use GetAllMessages() method of the queue and iterate through each message and further process them.
References:
a. MSMQ FAQs: http://www.microsoft.com/windowsserver2003/techinfo/overview/msmqfaq.mspx for more details.
b. MSMQ Complete Reference: http://www.microsoft.com/windowsserver2003/technologies/msmq/default.mspx
great article. thanks for the details.